While a lot of people are rewriting entire sites, in our industry, incremental releases are much easier to handle. One succesful method has been to create small stand-alone website that are simply hosted in a directory of the existing website.
For example, if you have a directory called “Purchasing”, where you have a PO list, PO Editor, Vendor List and a Vendor Editor – and all the subpages and widgets that they entail, you can simply make a new Angular Website to handle just those items, then compile it and run it in that directory.
This sounds easy, but Angular is finicky. I’m going to try to cover a few things here that are critical. There is a lot more and you all have your own favorite ways to do things, but this has been the absolute easiest for us.
Critical Points
- Setting up a development environment
- Enabling deep linking into that site on a website hosted by IIS
- Publishing to a remote server
Setup for Developing
I use Visual Studio 2020 for .Net development, but I use VS Code for Angular.
Normally, you will have your big website open locally and you’re running it via the Visual Studio engine in the browser. Here’s one that I wrote decades ago when .Net WebForms was the hot thing. (yikes!)
Now, (generally) your directory structure is just your website unless you’re using routing or MVC, but you get the idea. IIS on the server is going to route things to a directory.
In VS Code, you’ll have your angular app running, but instead of running it with ng serve or ng build, we’re going to use a custom build/watch command.
So, in your package.json, we’re going to add a custom command like this:
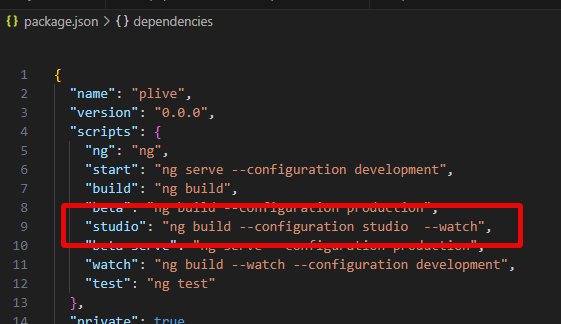
Then we need to define this “studio” configuration in your angular.json file.
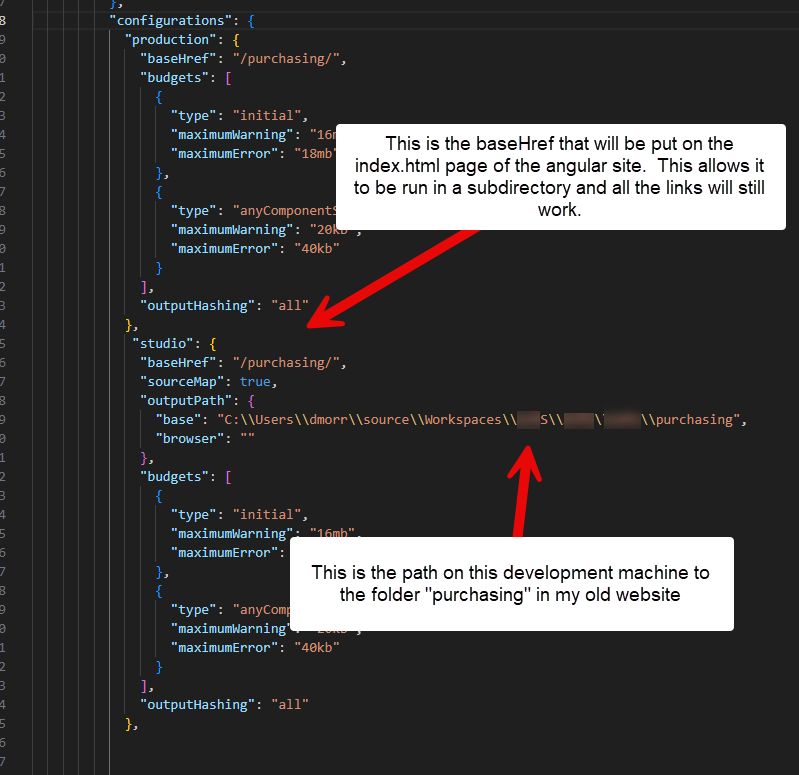
So now, typing “npm run studio” will compile the angular site into the folder called “purchasing” in my big old website. Since I’m running it in “watch” mode, when I make changes, it updates that folder. Unlike ng serve, this doesn’t autorefresh the browser, so you need to make your change, then refresh the browser manually.
At this point, you should have your main site running locally and browsing to that purchasing directory should take you to the angular website.
Deep Linking?
This is a common gotcha, but since you’re going to be on a windows server with IIS, the normal scripts to say “please use index.html for everything” doesn’t work. You need to include a web.config into the directory. IIS will read that and know what to do.
<?xml version="1.0" encoding="utf-8"?>
<configuration>
<system.webServer>
<rewrite>
<rules>
<!-- Redirect all requests to index.html -->
<rule name="Angular Routes" stopProcessing="true">
<match url=".*" />
<conditions logicalGrouping="MatchAll">
<!-- Ignore requests for files and directories -->
<add input="{REQUEST_FILENAME}" matchType="IsFile" negate="true" />
<add input="{REQUEST_FILENAME}" matchType="IsDirectory" negate="true" />
</conditions>
<action type="Rewrite" url="/purchasing/index.html" />
</rule>
</rules>
</rewrite>
</system.webServer>
<location path="index.html">
<system.webServer>
<httpProtocol>
<customHeaders>
<add name="Cache-Control" value="no-cache" />
</customHeaders>
</httpProtocol>
</system.webServer>
</location>
</configuration>
You’ll notice that we have a “Rewrite” command that sends it back to “/purchasing/index.html” which is the root page for our angular app. And additionally, we add a no-cache feature to ensure that your browser doesn’t keep saving the old one.
This is great, BUT you need to make sure it gets included in the build. I do it in the angular.json file like this:
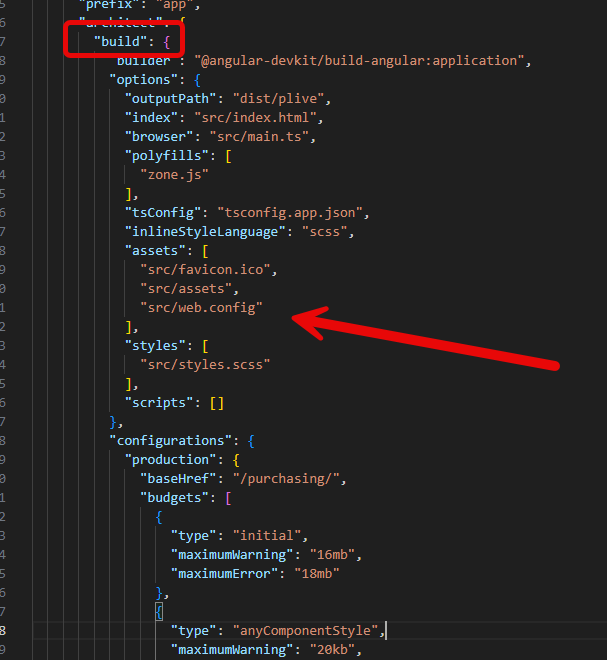
I keep the web.config file in the src directory of my angular project and I include it under the assets of the build section. As soon as it builds, it goes directly to the root of the angular app, in this case, in the /purchasing/ folder.
Publishing
I love continuous integration, BUT, that’s an entire subject of it’s own. Sometimes, quick and dirty is the way to go. Once it’s running locally and you can see it going, we are truly at the what-you-see-is-what-you-get. So, when you publish the entire site, you might go to the project, right click and choose Publish. That will take you to the publish screen:
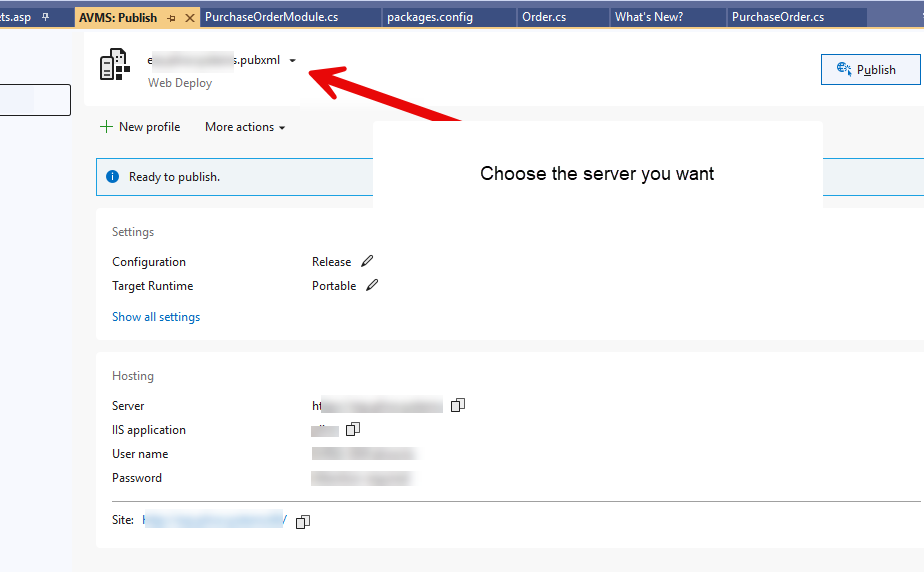
Now, that’s useless, right? Not exactly. All you need to do is choose from the options (or add a new one if you don’t already have it set up.)
Then go to your folder, right click on it, choose “Refresh” and then “Publish”.
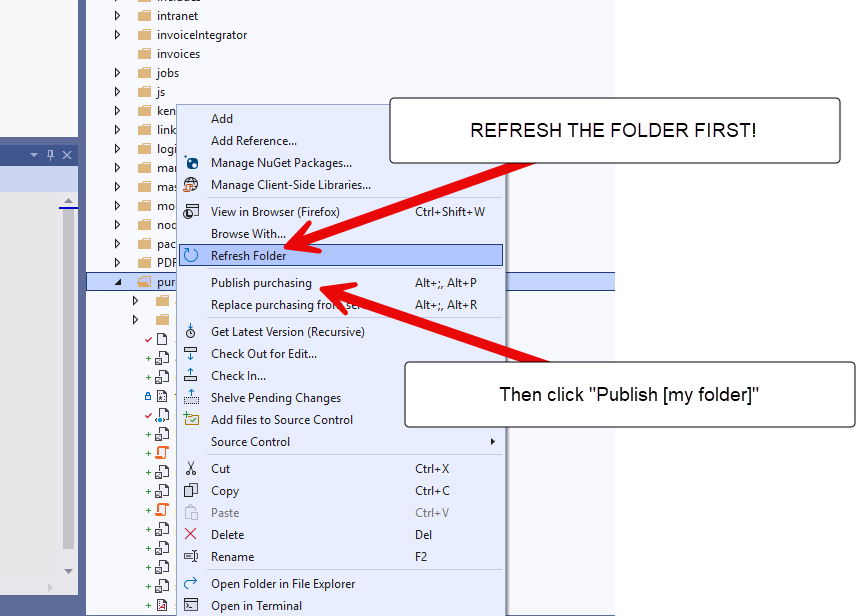
That will easily pop that folder up to your server, just like any other webpage. Once it’s there, it will be served exactly like any other angular website, but simply within that directory.
Conclusions
There are a million different ways to do this, and I actually use Azure Static Websites hosted through Azure FrontDoor to emulate this and still allow Continuous Integration and load balancing, etc… But, this is how you can really get started and move on from there.
As far as this idea of “microsites”, this is a tough decision to make. The reality that I run into quite often is *budgets*. Both time and money can suddenly dry up. If you decide to rebuild a system that took 10years to make, you’re setting yourself up for failure. You need to be able to stop, walk away, and have the site still be relevant in 2 years when whatever pandemic is over or whatever other emergency took your funding.
Another reality is the management model. It’s very hard to have a small development team be responsible for a gigantic website. However, it’s not hard to have 3 small development teams with their own websites and a common set of CSS and styles, sharing common API endpoints. Moreover, you can onboard a new candidate without overwhelming them with 2000000 pages of code.
For all small new sites, I’d always recommend Angular/C# as my favorite structure, but every time I’m handed a big mess from 1995, but 1000 people are using it for data-entry every day…. well, that’s when incremental development of microsites wins the prize every time.